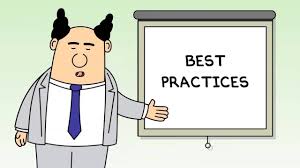
In my previous blog post, I explained the importance of writing testable code. In this post, I will explore some common best practices for unit testing in C#, focusing on key areas such as test structuring, mocking frameworks, test naming, and differentiating between unit and integration tests.
Follow the Arrange-Act-Assert (AAA) Pattern
The AAA pattern provides a structured approach for organizing unit tests. Follow these steps:
- Arrange: Set up the object to be tested and its dependencies.
- Act: Perform the action to be tested.
- Assert: Verify that the action led to the expected result.
Using the AAA pattern enhances readability and maintainability of your tests.
using Microsoft.VisualStudio.TestTools.UnitTesting;
namespace MyApp.Tests
{
[TestClass]
public class CalculatorTests
{
[TestMethod]
public void Add_GivenTwoIntegers_ReturnsTheirSum()
{
// Arrange
var calculator = new Calculator();
int number1 = 2;
int number2 = 3;
// Act
int result = calculator.Add(number1, number2);
// Assert
Assert.AreEqual(5, result, "The addition of 2 and 3 should equal 5");
}
}
}
Use Mocking Frameworks Wisely
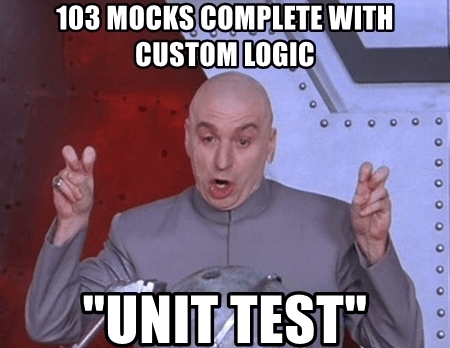
Mocking frameworks, like Moq, allow the creation of mock implementations of dependencies. However, use them judiciously to avoid potential pitfalls:
- False Sense of Security: Over-mocking can lead to tests passing despite real-world failures.
- Brittle Tests: Unmet mock expectations due to code changes can cause unnecessary test failures.
- Overemphasis on Implementation Details: Too many mocks may shift focus from testing behavior to testing implementation.
- Increased Complexity: Tests with excessive mocks become harder to understand and maintain.
- Balance the use of mocking frameworks to isolate code units effectively without introducing unnecessary complexity.
Write Meaningful Test Names
A good test name should describe what the test does and provide a clear idea of what went wrong in case of failure. Follow a convention like "MethodName_StateUnderTest_ExpectedBehavior" to improve test name clarity and simplicity.
Separate Unit and Integration Tests
Distinguish between unit tests and integration tests to ensure effective testing and maintainable code.
- Unit Tests: Focus on testing individual components in isolation, with mocked or stubbed dependencies. They are fast, cheap, and provide valuable feedback on small code units.
- Integration Tests: Verify interactions and integration between components, including infrastructure. They have higher code coverage but can be more complex and fragile.
Separating these test types in separate projects or folders or namespaces allows developers to leverage the strengths of each approach while maintaining code quality.
Utilize Parametrized or Data-Driven Tests
Parametrized tests allow running the same test method with different inputs and expected outcomes. In C#, you can use parameterized tests or data-driven tests with frameworks like MSTest. These tests provide flexibility and code reuse.
[TestClass]
public class CalculatorTests
{
private Calculator _calculator;
[TestInitialize]
public void Setup()
{
_calculator = new Calculator();
}
[TestMethod]
public void Add_PositiveNumbers_CorrectSum()
{
AddAndVerify(1, 1, 2);
AddAndVerify(2, 2, 4);
AddAndVerify(3, 3, 6);
}
[TestMethod]
public void Add_NegativeNumbers_CorrectSum()
{
AddAndVerify(-1, -1, -2);
AddAndVerify(-2, -2, -4);
AddAndVerify(-3, -3, -6);
}
private void AddAndVerify(int a, int b, int expected)
{
// Arrange - setup is done before this
// Act
int result = _calculator.Add(a, b);
// Assert
Assert.AreEqual(expected, result);
}
By applying these best practices, you can write robust, reliable, and maintainable unit tests in C#. The Arrange-Act-Assert pattern brings clarity and structure to your tests. Using mocking frameworks wisely, writing meaningful test names, separating unit and integration tests, and leveraging parametrized or data-driven tests enhance the effectiveness and efficiency of your test suite. Unit testing is an essential tool for building high-quality software, and following these best practices will greatly contribute to your success.